Built in providers
SuperTokens currently supports the following providers, but you can also add your own custom provider:
- Apple (
thirdPartyId: "apple"
) - Discord (
thirdPartyId: "discord"
) - Facebook (
thirdPartyId: "facebook"
) - Github (
thirdPartyId: "github"
) - Google (
thirdPartyId: "google"
) - LinkedIn (
thirdPartyId: "linkedin"
) - Twitter (
thirdPartyId: "twitter"
)
#
Step 1: Frontend setup- ReactJS
- Angular
- Vue
Import and all the built in providers that you wish to show in the UI as shown below.
// this goes in the auth route config of your frontend app (once the pre built UI script has been loaded)
(window as any).supertokensUIInit("supertokensui", {
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
(window as any).supertokensUIThirdParty.init({
signInAndUpFeature: {
providers: [
(window as any).supertokensUIThirdParty.Github.init(),
(window as any).supertokensUIThirdParty.Google.init(),
(window as any).supertokensUIThirdParty.Facebook.init(),
(window as any).supertokensUIThirdParty.Apple.init(),
],
// ...
},
// ...
}),
// ...
]
});
Import and all the built in providers that you wish to show in the UI as shown below.
import SuperTokens from "supertokens-auth-react";
import ThirdParty, {Google, Github, Facebook, Apple} from "supertokens-auth-react/recipe/thirdparty";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
ThirdParty.init({
signInAndUpFeature: {
providers: [
Github.init(),
Google.init(),
Facebook.init(),
Apple.init(),
],
// ...
},
// ...
}),
// ...
]
});
Import and all the built in providers that you wish to show in the UI as shown below.
// this goes in the auth route config of your frontend app (once the pre built UI script has been loaded)
(window as any).supertokensUIInit("supertokensui", {
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
(window as any).supertokensUIThirdParty.init({
signInAndUpFeature: {
providers: [
(window as any).supertokensUIThirdParty.Github.init(),
(window as any).supertokensUIThirdParty.Google.init(),
(window as any).supertokensUIThirdParty.Facebook.init(),
(window as any).supertokensUIThirdParty.Apple.init(),
],
// ...
},
// ...
}),
// ...
]
});
#
Changing the button styleOn the frontend, you can provide a button component to the in built providers defining your own UI. The component you add will be clickable by default.
- ReactJS
- Angular
- Vue
caution
This is not possible for non react apps at the moment. Please use custom UI instead for the sign in form.
import SuperTokens from "supertokens-auth-react";
import ThirdParty, {Google, Github, Facebook, Apple} from "supertokens-auth-react/recipe/thirdparty";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
ThirdParty.init({
signInAndUpFeature: {
providers: [
Github.init({
buttonComponent: (props: {name: string}) => <div></div>
}),
Google.init({
buttonComponent: (props: {name: string}) => <div></div>
}),
Facebook.init({
buttonComponent: (props: {name: string}) => <div></div>
}),
Apple.init({
buttonComponent: (props: {name: string}) => <div></div>
}),
],
// ...
},
// ...
}),
// ...
]
});
caution
This is not possible for non react apps at the moment. Please use custom UI instead for the sign in form.
#
Step 2: Adding providers config to the backendYou should add all the built in providers to the providers
array during the init
function call on the backend. At a minimum, you will require the client ID and secret (unless the provider supports PKCE flow), but you can also change our default behaviour for any of the in built providers.
- Dashboard
- NodeJS
- GoLang
- Python
- cURL
Important
import SuperTokens from "supertokens-node";
import ThirdParty from "supertokens-node/recipe/thirdparty";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
supertokens: {
connectionURI: "...",
},
recipeList: [
ThirdParty.init({
signInAndUpFeature: {
providers: [
{
config: {
thirdPartyId: "google",
clients: [{
clientId: "TODO: GOOGLE_CLIENT_ID",
clientSecret: "TODO: GOOGLE_CLIENT_SECRET"
}]
},
},
{
config: {
thirdPartyId: "github",
clients: [{
clientId: "TODO: GITHUB_CLIENT_ID",
clientSecret: "TODO: GITHUB_CLIENT_SECRET"
}]
},
},
{
config: {
thirdPartyId: "facebook",
clients: [{
clientId: "TODO: FACEBOOK_CLIENT_ID",
clientSecret: "TODO: FACEBOOK_CLIENT_SECRET"
}]
},
},
]
}
})
]
});
- Make sure that the above configurations for
"CLIENT_SECRET"
are stored in your environment variables and not directly in your source code files.
import (
"github.com/supertokens/supertokens-golang/recipe/thirdparty"
"github.com/supertokens/supertokens-golang/recipe/thirdparty/tpmodels"
"github.com/supertokens/supertokens-golang/supertokens"
)
func main() {
supertokens.Init(supertokens.TypeInput{
RecipeList: []supertokens.Recipe{
thirdparty.Init(&tpmodels.TypeInput{
SignInAndUpFeature: tpmodels.TypeInputSignInAndUp{
Providers: []tpmodels.ProviderInput{
{
Config: tpmodels.ProviderConfig{
ThirdPartyId: "google",
Clients: []tpmodels.ProviderClientConfig{
{
ClientID: "TODO: GOOGLE_CLIENT_ID",
ClientSecret: "TODO: GOOGLE_CLIENT_SECRET",
},
},
},
},
{
Config: tpmodels.ProviderConfig{
ThirdPartyId: "github",
Clients: []tpmodels.ProviderClientConfig{
{
ClientID: "TODO: GITHUB_CLIENT_ID",
ClientSecret: "TODO: GITHUB_CLIENT_SECRET",
},
},
},
},
{
Config: tpmodels.ProviderConfig{
ThirdPartyId: "facebook",
Clients: []tpmodels.ProviderClientConfig{
{
ClientID: "TODO: FACEBOOK_CLIENT_ID",
ClientSecret: "TODO: FACEBOOK_CLIENT_SECRET",
},
},
},
},
},
},
}),
},
})
}
- Make sure that the above configurations for
"CLIENT_SECRET"
are stored in your environment variables and not directly in your source code files.
from supertokens_python import init, InputAppInfo
from supertokens_python.recipe import thirdparty
from supertokens_python.recipe.thirdparty import ProviderInput, ProviderConfig, ProviderClientConfig, SignInAndUpFeature
init(
app_info=InputAppInfo(api_domain="...", app_name="...", website_domain="..."),
framework='...',
recipe_list=[
thirdparty.init(
sign_in_and_up_feature=SignInAndUpFeature(
providers=[
ProviderInput(
config=ProviderConfig(
third_party_id="google",
clients=[
ProviderClientConfig(
client_id="GOOGLE_CLIENT_ID",
client_secret="GOOGLE_CLIENT_SECRET",
),
],
),
),
ProviderInput(
config=ProviderConfig(
third_party_id="facebook",
clients=[
ProviderClientConfig(
client_id="FACEBOOK_CLIENT_ID",
client_secret="FACEBOOK_CLIENT_SECRET",
),
],
),
),
ProviderInput(
config=ProviderConfig(
third_party_id="github",
clients=[
ProviderClientConfig(
client_id="GITHUB_CLIENT_ID",
client_secret="GITHUB_CLIENT_SECRET",
),
],
),
),
]
)
)
]
)
- Make sure that the above configurations for
"CLIENT_SECRET"
are stored in your environment variables and not directly in your source code files.
- Single app setup
- Multi app setup
curl --location --request PUT '/public/recipe/multitenancy/config/thirdparty' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"config": {
"thirdPartyId": "google",
"clients": [
{
"clientId": "...",
"clientSecret": "..."
}
]
}
}'
curl --location --request PUT '/public/recipe/multitenancy/config/thirdparty' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"config": {
"thirdPartyId": "github",
"clients": [
{
"clientId": "...",
"clientSecret": "..."
}
]
}
}'
curl --location --request PUT '/public/recipe/multitenancy/config/thirdparty' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"config": {
"thirdPartyId": "facebook",
"clients": [
{
"clientId": "...",
"clientSecret": "..."
}
]
}
}'
curl --location --request PUT '/public/recipe/multitenancy/config/thirdparty' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"config": {
"thirdPartyId": "google",
"clients": [
{
"clientId": "...",
"clientSecret": "..."
}
]
}
}'
curl --location --request PUT '/public/recipe/multitenancy/config/thirdparty' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"config": {
"thirdPartyId": "github",
"clients": [
{
"clientId": "...",
"clientSecret": "..."
}
]
}
}'
curl --location --request PUT '/public/recipe/multitenancy/config/thirdparty' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"config": {
"thirdPartyId": "facebook",
"clients": [
{
"clientId": "...",
"clientSecret": "..."
}
]
}
}'
You can also add providers using the Dashboard. Access the public tenant from the tenant management page and then add the providers in the Social/Enterprise Providers section.
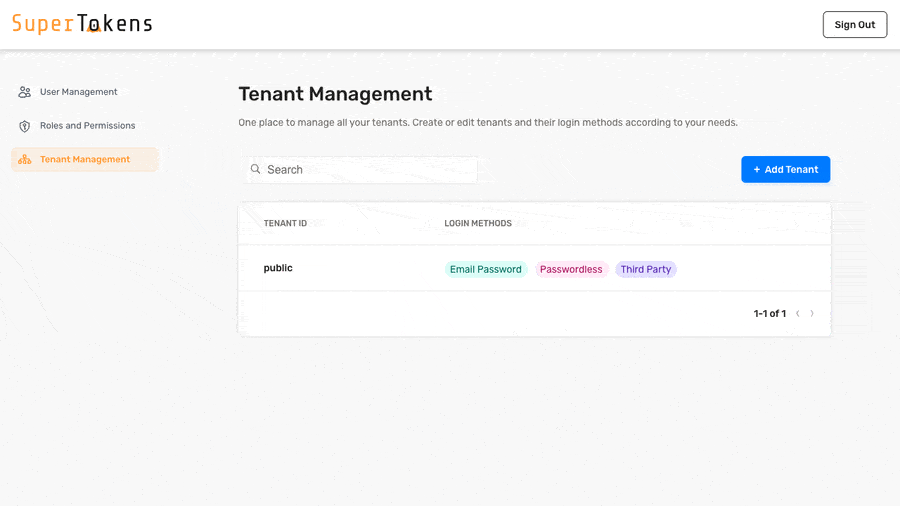
- You can see all the configs available for each of our built in providers over here
#
Setting OAuth ScopesIf you would like to add additional OAuth Scopes when accessing your third party provider, you can do so by adding them to the config when initializing the backend SDK.
For example if you are using Google as your third party provider, you can add an additional scope as follows:
- NodeJS
- GoLang
- Python
- Other Frameworks
Important
import SuperTokens from "supertokens-node";
import ThirdParty from "supertokens-node/recipe/thirdparty";
SuperTokens.init({
supertokens: {
connectionURI: "...",
},
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
ThirdParty.init({
signInAndUpFeature: {
providers: [
{
config: {
thirdPartyId: "google",
clients: [{
clientId: "TODO: GOOGLE_CLIENT_ID",
clientSecret: "TODO: GOOGLE_CLIENT_SECRET",
scope: ["scope1", "scope2"]
}]
}
}
]
}
})
]
});
import (
"github.com/supertokens/supertokens-golang/recipe/thirdparty"
"github.com/supertokens/supertokens-golang/recipe/thirdparty/tpmodels"
"github.com/supertokens/supertokens-golang/supertokens"
)
func main() {
supertokens.Init(supertokens.TypeInput{
RecipeList: []supertokens.Recipe{
thirdparty.Init(&tpmodels.TypeInput{
SignInAndUpFeature: tpmodels.TypeInputSignInAndUp{
Providers: []tpmodels.ProviderInput{
{
Config: tpmodels.ProviderConfig{
ThirdPartyId: "google",
Clients: []tpmodels.ProviderClientConfig{
{
ClientID: "TODO: GOOGLE_CLIENT_ID",
ClientSecret: "TODO: GOOGLE_CLIENT_SECRET",
Scope: []string{
"scope1", "scope2",
},
},
},
},
},
},
},
}),
},
})
}
from supertokens_python import init, InputAppInfo
from supertokens_python.recipe import thirdparty
from supertokens_python.recipe.thirdparty import ProviderInput, ProviderConfig, ProviderClientConfig, SignInAndUpFeature
init(
app_info=InputAppInfo(api_domain="...", app_name="...", website_domain="..."),
framework='...',
recipe_list=[
thirdparty.init(
sign_in_and_up_feature=SignInAndUpFeature(
providers=[
ProviderInput(
config=ProviderConfig(
third_party_id="google",
clients=[
ProviderClientConfig(
client_id="GOOGLE_CLIENT_ID",
client_secret="GOOGLE_CLIENT_SECRET",
scope=["scope1", "scope2"]
),
],
),
),
]
)
)
]
)
important
Along with your custom scopes, you also need to add scopes that ask for the user's email and its verification status. For example with Google, this scope is called "https://www.googleapis.com/auth/userinfo.email"