Paid Feature
This is a paid feature.
You can click on the Enable Paid Features button on our dashboard, and follow the steps from there on. Once enabled, this feature is free on the provided development environment.
Unified Login
If your use case involves having to authenticate different types of applications using a common Authorization Server you can achieve this by using SuperTokens.
The implementation will involve configuring an OAuth2 Provider that will authenticate and authorize your existing clients.
#
When to use Unified LoginThere are a couple of common scenarios in which the Unified Login approach can be used. They are outlined below and we provide separate guides for each of them.
- If you have multiple frontend clients that connect to different backends
- If you have multiple frontend clients connecting to the same backend
- If you want to reuse your website login for desktop and mobile apps
For these specific instances we expose recipes that allow you to complete your setup.
caution
There are a few limitations with the OAuth2 recipes:
- It is only available for the SuperTokens Managed Service. This feature is not included in the Self-Hosted version.
- Only the
supertokens-node
backend SDK supports it as of yet. So if you are using ourgolang
orpython
SDK, you will have to wait for the next releases or configure a separate NodeJS Authorization Service. - We do not support magic link based login. However, you can switch to
email
/SMS
OTP instead. This method offers the same level of security. - Step Up Authentication is not available out of the box. You will have to use customizations in order to support the flow.
#
OAuth2 BasicsBefore we explore the guides let's first recap some common terms and concepts that are the base of the framework. We will use them throughout the next pages.
OAuth2, Open Authorization, is an industry-standard authorization framework that enables third-party applications to obtain limited access to a user's resources without exposing their credentials.
#
RolesIn OAuth, roles define the different responsibilities of entities involved in the process of granting and obtaining access to protected resources. There are four roles defined in the specification:
#
Resource OwnerThe Resource Owner is an entity capable of granting access to a protected resource. In most cases, this is an actual person that uses an application.
#
ClientAn OAuth 2.0 Client is an application that wants to access protected resources. In order to do so it needs to get an OAuth2 Access Token from the Authorization Server. With that token the client can perform authorized operations on behalf of the Resource Owner.
The term client does not imply any particular implementation characteristics (e.g. whether the application executes on a server, a desktop, or other devices).
#
Resource ServerThe server hosting the protected resources, capable of accepting and responding to protected resource requests using OAuth2 Access Tokens. Some real-world examples in this case would be things like:
- A file storage service that allows users to access only their files
- A social media application that allows users to access only posts from their friends
- A chat app that shows only messages from conversations in which the user is a participant
#
Authorization ServerThe server issuing OAuth2 Access Tokens to the Client after successfully authenticating the Resource Owner.
#
TokensTokens are strings that represent the authorization issued to the Client. They are mainly used to access to protected resources, on behalf of the Resource Owner. At the same time, tokens can be used to get more information about who the owner is.
#
OAuth2 Access TokenThis is the main token that is used to provide temporary access to protected resources. The OAuth2 Access Token is meant to be read and validated only by the Resource Server.
info
This token is different from the SuperTokens Session Access Token. The latter is used in the OAuth 2.0 authentication flows to maintain a session between the authorization frontend and the authorization backend server.
#
OAuth2 Refresh TokenA token that can be used to get a new OAuth2 Access Token when the current one has expired. Using the refresh token will not require the user to re-authenticate.
info
This token is different from the SuperTokens Session Refresh Token. The latter is used in the OAuth 2.0 authentication flows to maintain a session between the authorization frontend and the authorization backend server.
#
ID TokenThis token provides identity information about the Resource Owner. Unlike OAuth2 Access Tokens, the ID Tokens are meant to be read only by the Client.
#
ScopesScopes define the range of access that the Client is requesting on behalf of the Resource Owner. They specify what portions of the Resource Owner’s data the Client can access and what actions it can perform.
For example, when a user grants a web application permission to read their email, the application might request the email
scope.
In a general authentication flow scopes get used in the following way:
- When the Client gets created a series of scopes will be specified to the Authorization Server
- The Autorization Server authenticates the Resource Owner and uses the scopes to generate an OAuth2 Access Token.
- The Resource Server will check the scopes of the OAuth2 Access Token and will only allow the requested actions.
#
Authorization FlowsThe OAuth 2.0 protocol defines several flows to accommodate different use cases. They are a set of steps an OAuth Client has to perform in order to obtain an access token.
Our implementation supports the following flow types:
Authorization Code Grant#
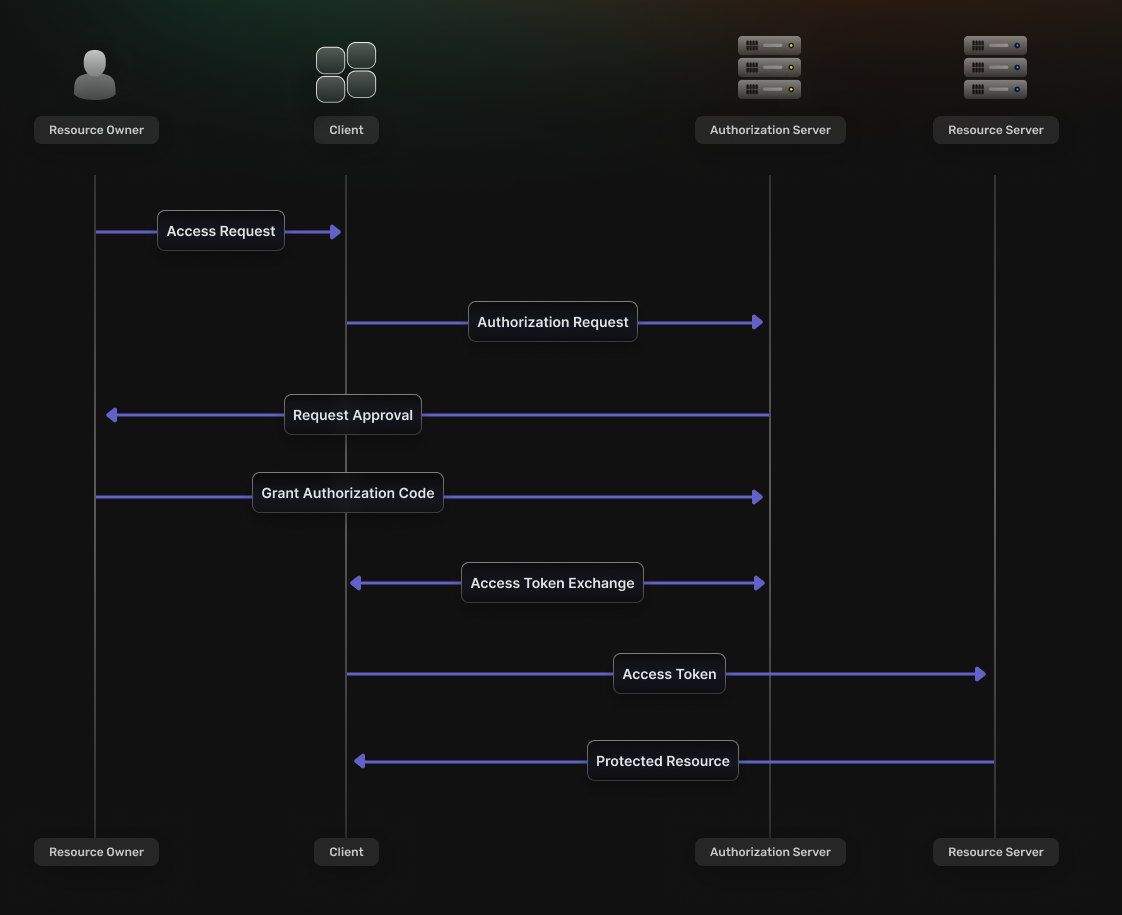
This flow is best suited for scenarios that involve web applications. It consists of the following steps:
- The Client redirects the Resource Owner to the Authorization Server’s authorization endpoint.
- If the Resource Owner grants permission, the Authorization Server redirects their browser back to the specified Redirect URI and includes an Authorization Code as a query parameter.
- The Client then sends a request to the Authorization Server’s token endpoint, including the Authorization Code.
- The Authorization Server verifies the information sent by the Client and, if valid, issues an OAuth2 Access Token.
- The token can now be used to make requests to the Resource Server to access the protected resources on behalf of the Resource Owner.
#
Authorization CodeAn Authorization Code is a short-lived code that the Authorization Server provides to the Client, via a Redirect URI, after authorization has been granted. This code then gets exchanged for an OAuth2 Access Token. The Authorization Code flow enhances security by keeping tokens out of the user-agent and letting the Client manage the backend communication with the Authorization Server.
#
Proof Key for Code Exchange (PKCE)In order to prevent CSRF and code injection attacks, the Authorization Code flow can be enhanced with PKCE.
At the beginning of the authentication flow the Client will generate a random string called a code verifier. This gets used to ensure that, even if the Authorization Code is intercepted, it cannot be exchanged for a token without also including the initial code.
Client Credentials#
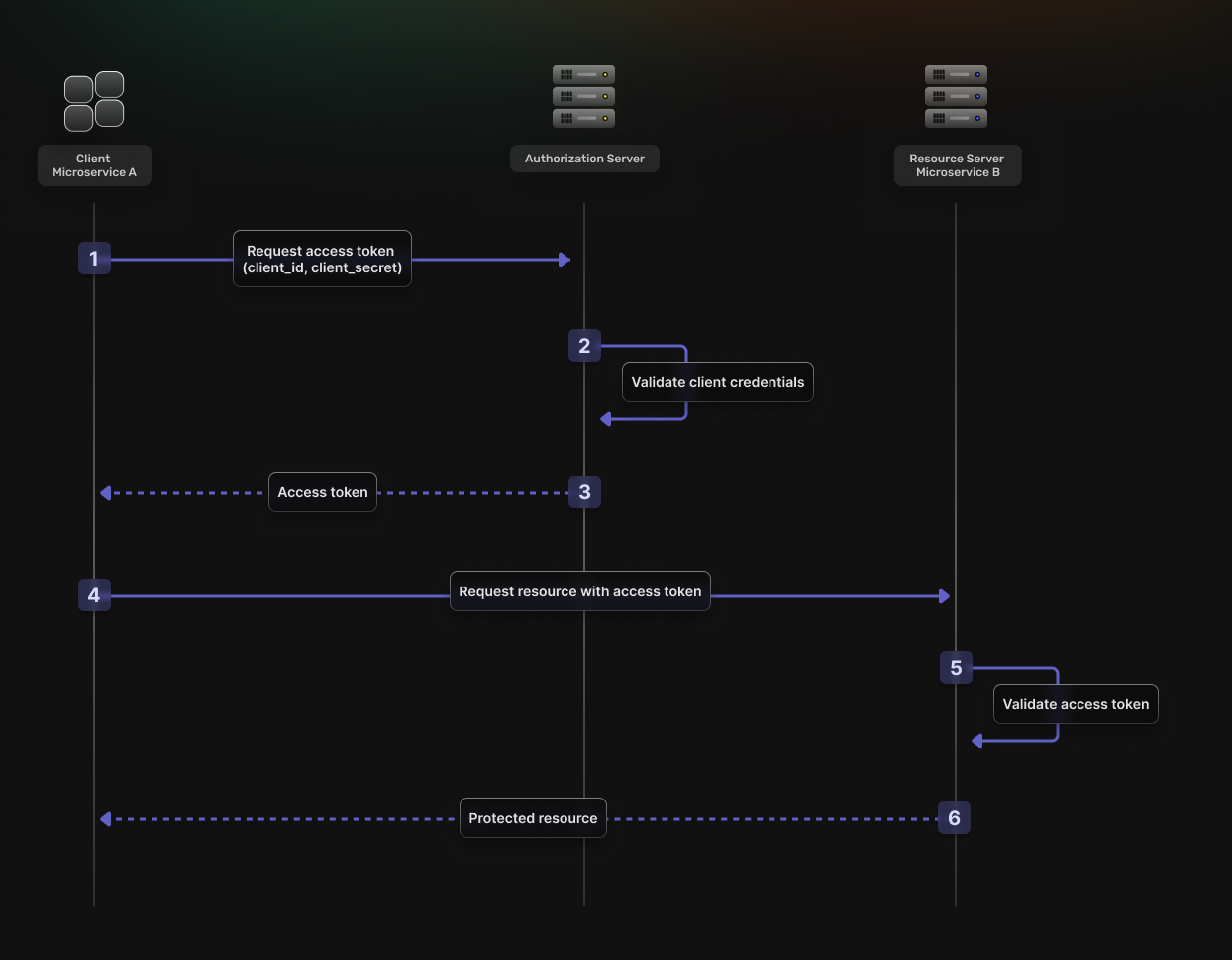
This flow is best suited for machine-to-machine (M2M) interactions where there is no end-user. It consists of the following steps:
- The Client authenticates with the Authorization Server using its own credentials.
- The Authorization Server verifies the credentials.
- The Authorization Server returns an OAuth2 Access Token.
- The Client uses the OAuth2 Access Token to access protected resources.
- The Resource Server validates the OAuth2 Access Token.
- If the validation is successful, the Resource Server returns the requested resources.