Changing Style via CSS
What type of UI are you using?
Updating the CSS allows you to change the UI of our components to meet your needs.
This section will guide you through an example of updating the look of buttons. Note that the process can be applied to update any HTML tag from within SuperTokens components.
Global style changes
How to make changes
First, let's open our website at /auth
. The Sign-in widget should show up. Let's use the browser console to find out the class name that we'd like to overwrite.
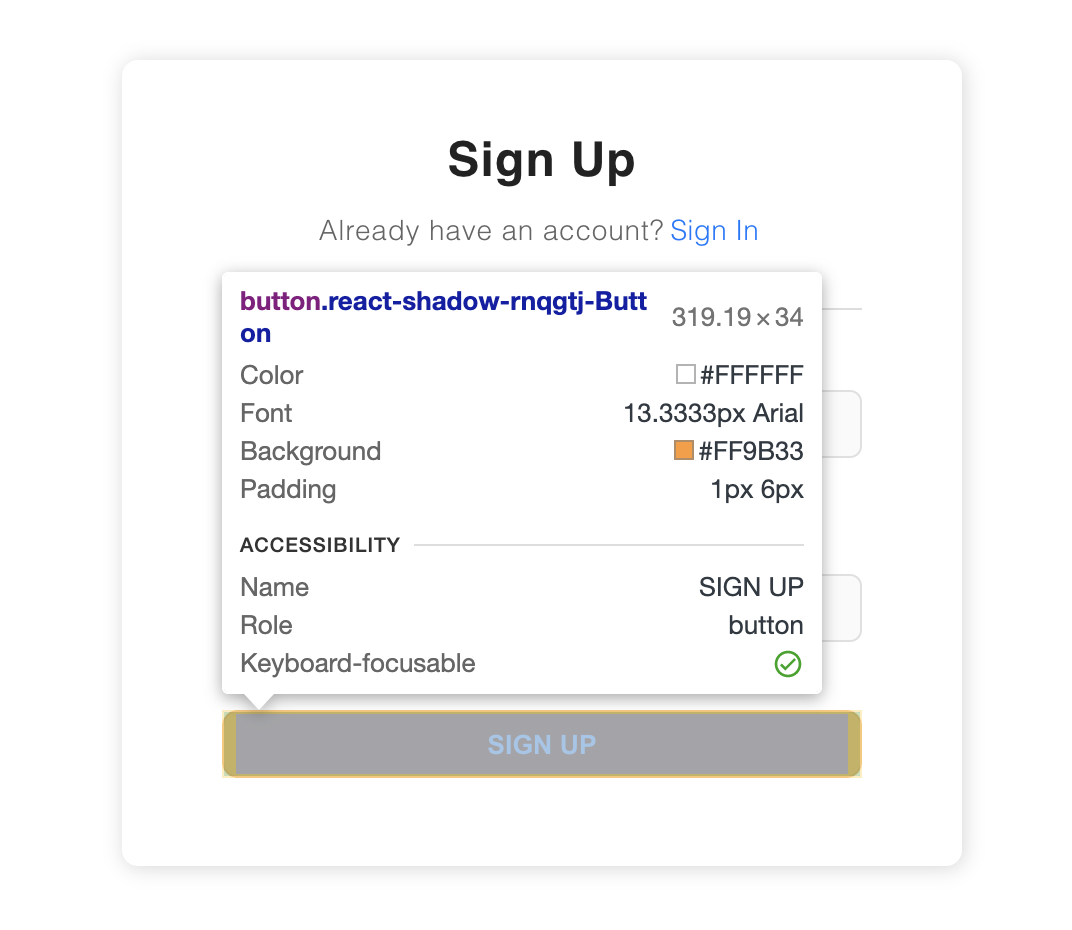
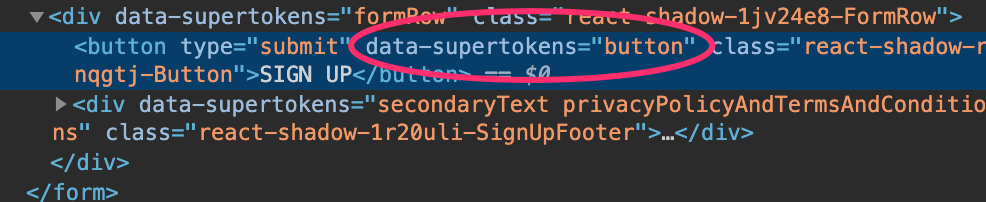
Each stylable components contains data-supertokens
attributes (in our example data-supertokens="button"
).
Let's customize elements with the button
attribute. The syntax for styling is plain CSS.
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
style: `
[data-supertokens~=button] {
background-color: #252571;
border: 0px;
width: 30%;
margin: 0 auto;
}
`,
recipeList: [ /* ... */]
});
The above will result in:
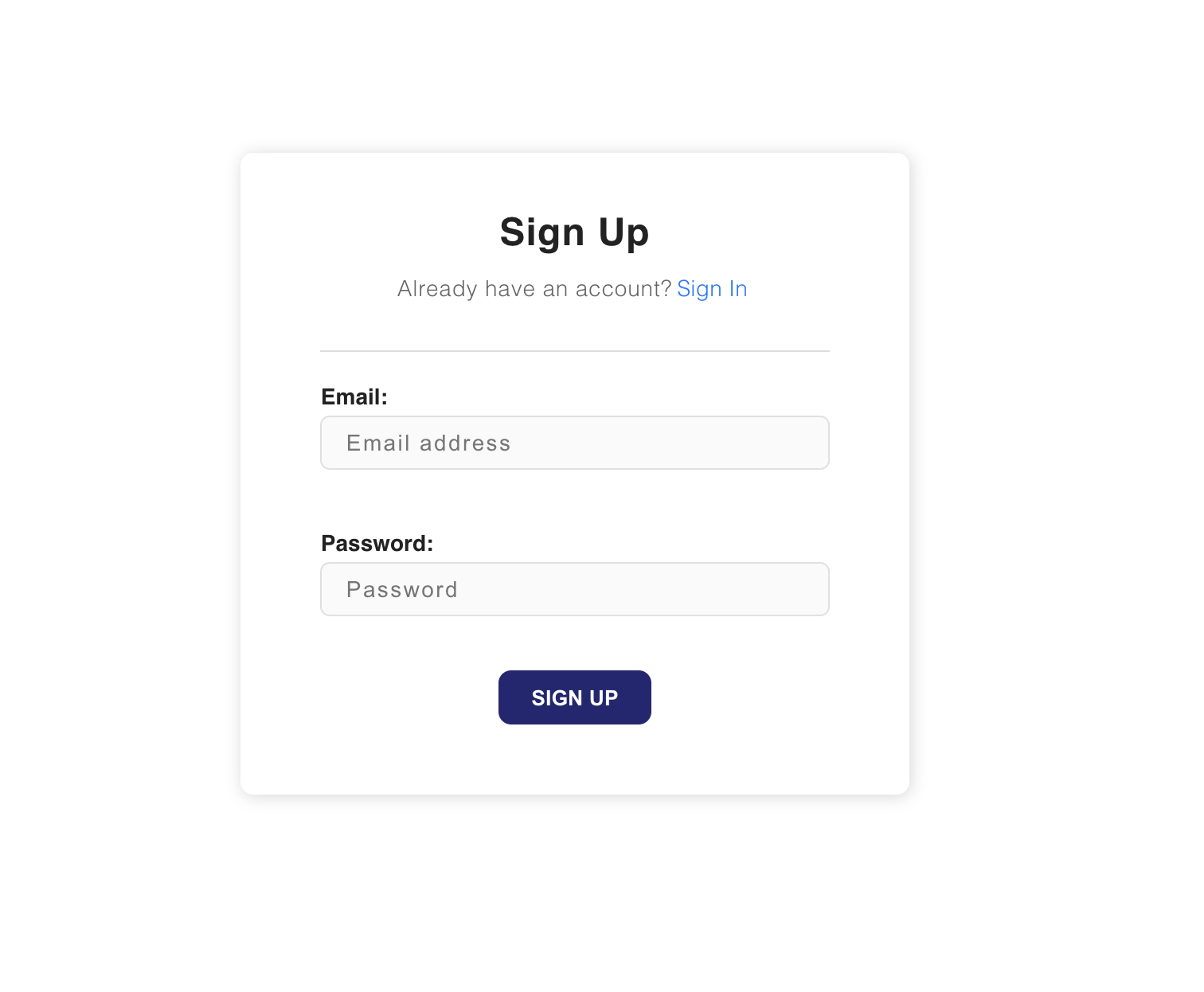
Changing fonts
By default, SuperTokens uses the Arial
font. The best way to override this is to add a font-family
styling to the container
component in the recipe configuration.
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
style: `
[data-supertokens~=container] {
font-family: cursive;
}
`,
recipeList: [ /* ... */]
});
Using media queries
You may want to have different CSS for different viewports
. This can be achieved via media queries like this:
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "...",
},
style: `
[data-supertokens~=button] {
background-color: #252571;
border: 0px;
width: 30%;
margin: 0 auto;
}
@media (max-width: 440px) {
[data-supertokens~=button] {
width: 90%;
}
}
`,
recipeList: [ /* ... */],
});
Customising the Sign-Up / Sign-In form
These are the screens shown when the user tries to log in or sign up for the application.
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
style: `[data-supertokens~=authPage] {
...
}`,
recipeList: [ /* ... */]
});
Customising the Password Reset forms
Send password reset email form
This form is shown when the user clicks on "forgot password" in the sign in form.
import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "...",
},
recipeList: [
EmailPassword.init({
resetPasswordUsingTokenFeature: {
enterEmailForm: {
style: ` ... `
}
}
}),
Session.init()
]
});
Submit new password form
This screen is shown when the user clicks the password reset link on their email - to enter their new password
import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "...",
},
recipeList: [
EmailPassword.init({
resetPasswordUsingTokenFeature: {
submitNewPasswordForm: {
style: ` ... `
}
}
}),
Session.init()
]
});