important
Please select a recipe to get started
#
Authentication MethodsEmail password with Social / Enterprise Login
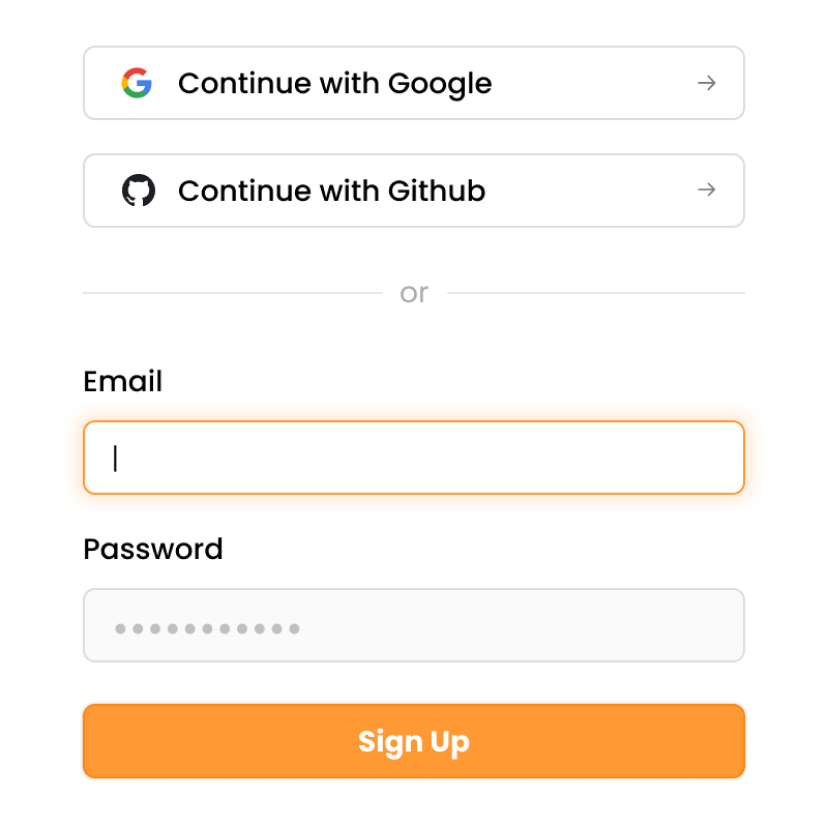
Passwordless
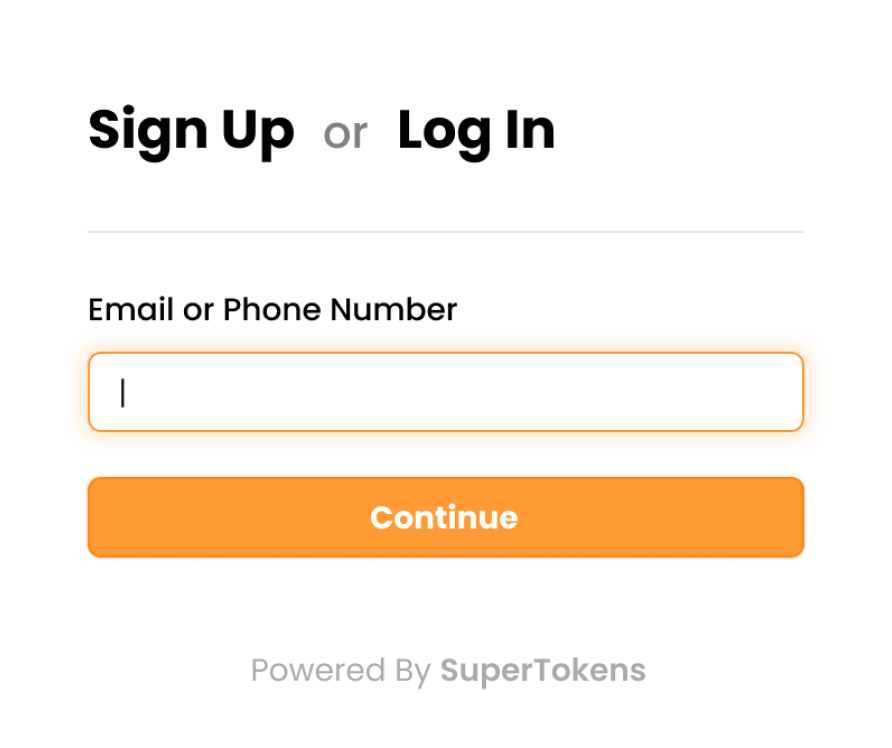
Email password Login
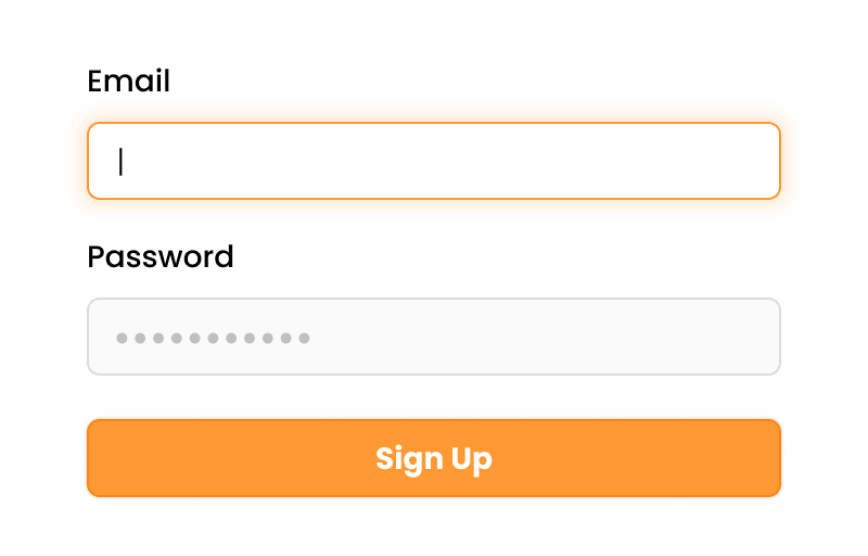
Social / Enterprise Login with Passwordless
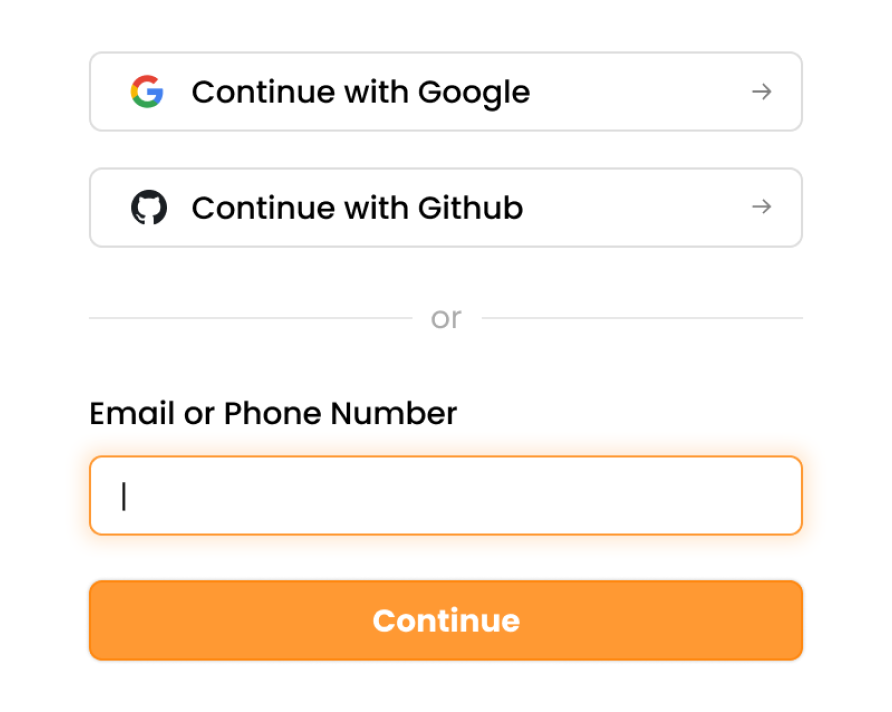
Social / Enterprise Login
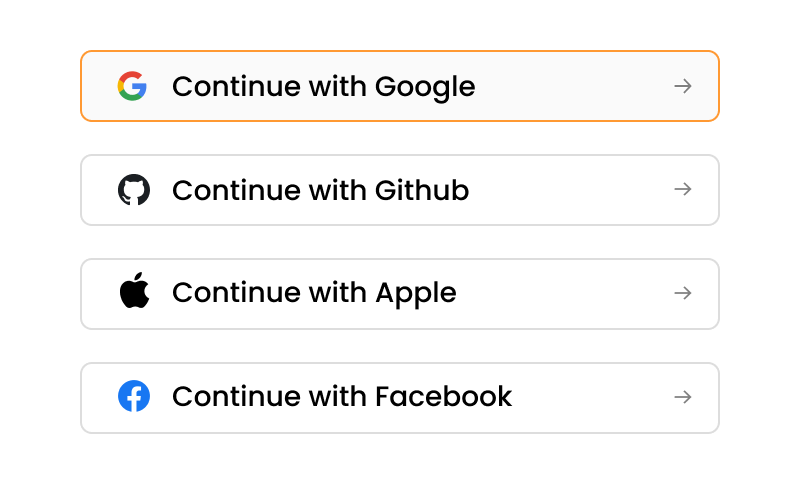
Phone Password Login
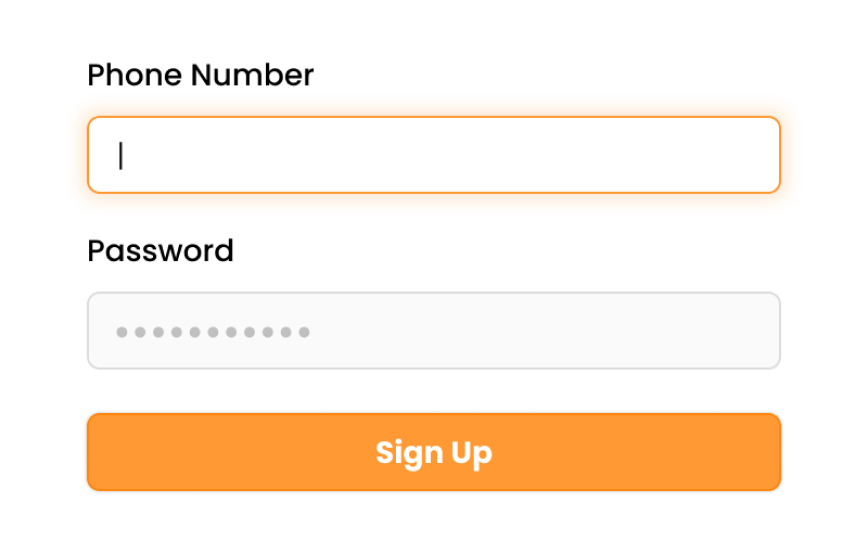
#
Add-onsUserRoles (RBAC)
Multi factor Authentication
Microservice Authentication
Session Management
User Management Dashboard
Multi tenancy / organizations