Introduction
Authenticate users with email/password, customize UI, and explore SDK integration options.
Overview
The Email/Password recipe
provides a way of authenticating users with basic credentials.
You can use it out of the box, with the Pre-Built UI, or implement your own interface through the available SDKs.
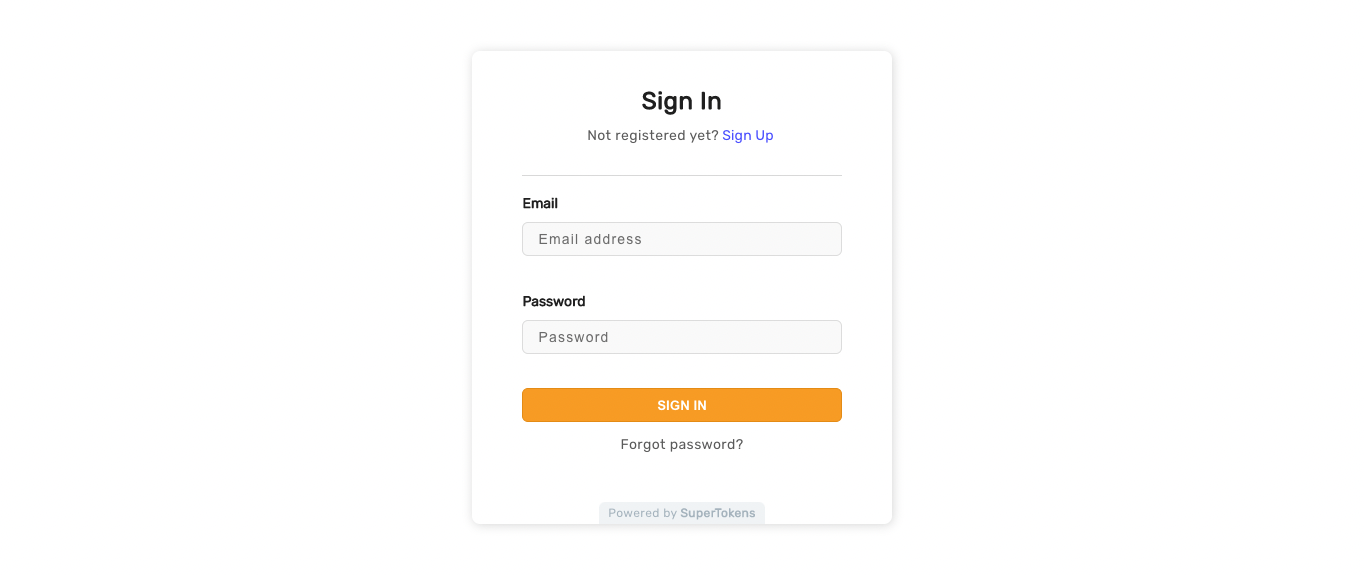
Getting started
You can either follow the quickstart tutorial or use the CLI
tool to generate an example app that shows you how the recipe works.
Quickstart
Go through a quick tutorial that shows you how to add the Email/Password recipe to your app.
Example Apps
Use the CLI to generate a boilerplate app that you can use as a starting point.
Customization
To adjust the functionality to fit your use case you can explore different sections from the documentation.
Sign In Form Customization
Adapt the look and feel of the pre-built sign in form.
Sign Up Form Customization
Adapt the look and feel of the pre-built sign up form.
Hooks and Overrides
Add custom logic after the user logs in or signs up.
Password Hashing
Read which hashing algorithms you can use and how to use them.
Username Login
Discover how you can implement an authentication flow that makes use of usernames instead of email addresses.