Introduction
Authenticate users with passwordless methods like Magic Links or One-Time Passwords.
Overview
The Passwordless recipe
provides a way of authenticating users through generated credentials like Magic Links or One-Time Passwords.
You can use it out of the box, with the Pre-Built UI, or implement your own interface through the available SDKs.
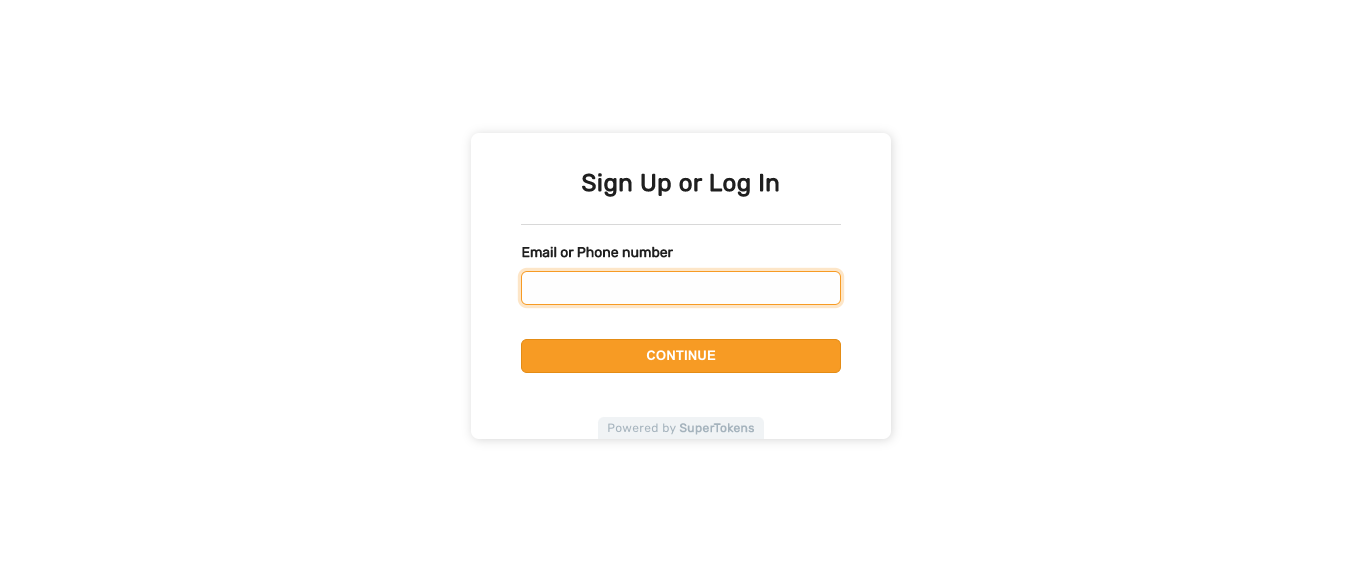
Getting started
You can either follow setup guide or use the CLI
tool to generate an example app that shows you how the recipe works.
Quickstart
Go through a quick tutorial that shows you how to add the Passwordless recipe to your app.
Example Apps
Use the CLI to generate a boilerplate app that you can use as a starting point.
Customization
To adjust the functionality to fit your use case you can explore different sections from the documentation.
Customize the Magic Link
Change how you create Magic Links.
One-Time Password (OTP) Customization
Change the format of the generated One-Time Password.
Hooks and Overrides
Add custom logic after the logs in or signs up.
Email Delivery
Customize how you deliver emails to your users.
SMS Delivery
Customize how you deliver SMS messages to your users.