Frontend Integration
#
Supported frameworksfor mobile apps
For mobile apps, please see the "Using your own UI" section (see left nav bar)
Automatic setup using CLI
Run the following command in your terminal.
npx create-supertokens-app@latest --recipe=emailpassword
Once this is done, you can skip Step (1) and (2) in this section (see left nav bar) and move directly to setting up the SuperTokens core (Step 3).
Or, you can manually integrate SuperTokens by following the steps below.
Manual setup steps below
#
1) Install- ReactJS
- Angular
- Vue
- Mobile
- Via NPM>=7
- Via NPM6
- Via Yarn
npm i -s supertokens-auth-react
npm i -s supertokens-auth-react supertokens-web-js
yarn add supertokens-auth-react supertokens-web-js
- Via NPM>=7
- Via NPM6
- Via Yarn
important
SuperTokens does not provide Angular components for pre built UI, but, there are two ways of implementing SuperTokens in your Angular frontend:
- Load SuperTokens's pre-built React UI components in your Angular app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react
important
SuperTokens does not provide Angular components for pre built UI, but, there are two ways of implementing SuperTokens in your Angular frontend:
- Load SuperTokens's pre-built React UI components in your Angular app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react supertokens-web-js
important
SuperTokens does not provide Angular components for pre built UI, but, there are two ways of implementing SuperTokens in your Angular frontend:
- Load SuperTokens's pre-built React UI components in your Angular app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
yarn add supertokens-auth-react supertokens-web-js
You will also need to update compilerOptions
in your tsconfig.json
file with the following:
"jsx": "react",
"allowSyntheticDefaultImports": true,
- Via NPM>=7
- Via NPM6
- Via Yarn
important
SuperTokens does not provide Vue components for pre built UI, but, there are two ways of implementing SuperTokens in your Vue frontend:
- Load SuperTokens's pre-built React UI components in your Vue app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react
important
SuperTokens does not provide Vue components for pre built UI, but, there are two ways of implementing SuperTokens in your Vue frontend:
- Load SuperTokens's pre-built React UI components in your Vue app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react supertokens-web-js
important
SuperTokens does not provide Vue components for pre built UI, but, there are two ways of implementing SuperTokens in your Vue frontend:
- Load SuperTokens's pre-built React UI components in your Vue app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
yarn add supertokens-auth-react supertokens-web-js
important
SuperTokens does not support pre-built UI for mobile frameworks. Please refer to the setup guide for custom UI to integrate SuperTokens in your app.
init
function#
2) Call the - ReactJS
- Angular
- Vue
- Mobile
In your App.js
file, import SuperTokens and call the init
function
import React from 'react';
import SuperTokens, { SuperTokensWrapper } from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
// learn more about this on https://supertokens.com/docs/emailpassword/appinfo
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
websiteDomain: "<YOUR_WEBSITE_DOMAIN>",
apiBasePath: "/auth",
websiteBasePath: "/auth",
},
recipeList: [
EmailPassword.init(),
Session.init()
]
});
/* Your App */
class App extends React.Component {
render() {
return (
<SuperTokensWrapper>
{/*Your app components*/}
</SuperTokensWrapper>
);
}
}
Before we initialize the supertokens-auth-react
SDK let's see how we will use it in our Angular app
Architecture
- The
supertokens-auth-react
SDK is responsible for rendering the login UI, handling authentication flows and session management. - Using
supertokens-auth-react
's pre-built UI does not increase application's bundle size. React is only added to authentication-related routes.
Creating the /auth
route
Use the Angular CLI to generate a new route
ng generate module auth --route auth --module app.module
In your
auth
component folder create a react componentsupertokensAuthComponent.tsx
. This component will be used to render appropriate UI when user visits the login page./app/auth/supertokensAuthComponent.tsximport * as React from "react";
import * as SuperTokens from "supertokens-auth-react";
import { canHandleRoute, getRoutingComponent } from "supertokens-auth-react/ui";
import { EmailPasswordPreBuiltUI } from 'supertokens-auth-react/recipe/emailpassword/prebuiltui';
class SuperTokensReactComponent extends React.Component {
override render() {
if (canHandleRoute([EmailPasswordPreBuiltUI])) {
return getRoutingComponent([EmailPasswordPreBuiltUI]);
}
return "Route not found";
}
}
export default SuperTokensReactComponent;Load
superTokensAuthComponent
in theauth
angular component/app/auth/auth.component.tsimport { AfterViewInit, Component, OnDestroy } from "@angular/core";
import * as React from "react";
import * as ReactDOM from "react-dom";
import SuperTokensReactComponent from "./supertokensAuthComponent.tsx";
@Component({
selector: "app-auth",
template: '<div [id]="rootId"></div>',
})
export class AuthComponent implements AfterViewInit, OnDestroy {
title = "angularreactapp";
public rootId = "rootId";
// We use the ngAfterViewInit lifecycle hook to render the React component after the Angular component view gets initialized
ngAfterViewInit() {
ReactDOM.render(React.createElement(SuperTokensReactComponent), document.getElementById(this.rootId));
}
// We use the ngOnDestroy lifecycle hook to unmount the React component when the Angular wrapper component is destroyed.
ngOnDestroy() {
ReactDOM.unmountComponentAtNode(document.getElementById(this.rootId) as Element);
}
}Initialize the
supertokens-auth-react
SDK in your angular app's root component. This will provide session management across your entire application./app/app.component.tsimport { Component } from "@angular/core";
import * as SuperTokens from "supertokens-auth-react";
import * as EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
websiteDomain: "<YOUR_WEBSITE_DOMAIN>",
apiBasePath: "/auth",
websiteBasePath: "/auth"
},
recipeList: [
EmailPassword.init(),
Session.init(),
],
});
@Component({
selector: "app-root",
template: "<router-outlet></router-outlet>",
})
export class AppComponent {
title = "<YOUR_APP_NAME>";
}
Before we initialize the supertokens-auth-react
SDK let's see how we will use it in our Vue app
Architecture
- The
supertokens-auth-react
SDK is responsible for rendering the login UI, handling authentication flows and session management. - Using
supertokens-auth-react
's pre-built UI does not increase application's bundle size. React is only added to authentication-related routes.
Creating the /auth
route
Create a new file
AuthView.vue
, this Vue component will be used to render the auth componentCreate a React component
Supertokens.tsx
. This will tell SuperTokens which UI to show when the user visits the login page./components/Supertokens.tsximport * as SuperTokens from "supertokens-auth-react";
import { canHandleRoute, getRoutingComponent } from "supertokens-auth-react/ui";
import { EmailPasswordPreBuiltUI } from 'supertokens-auth-react/recipe/emailpassword/prebuiltui';
function SuperTokensReactComponent(props: any) {
if (canHandleRoute([EmailPasswordPreBuiltUI])) {
return getRoutingComponent([EmailPasswordPreBuiltUI]);
}
return "Route not found";
}
export default SuperTokensReactComponent;Load
SuperTokensReactComponent
in theAuthView.vue
component/views/AuthView.vue<script lang="ts">
import * as React from "react";
import * as ReactDOM from "react-dom";
import SuperTokensReactComponent from "../components/Supertokens";
export default {
mounted: () => {
ReactDOM.render(
React.createElement(SuperTokensReactComponent as React.FC),
document.getElementById("authId")
);
}
};
</script>
<template>
<main>
<div id="authId" />
</main>
</template>Initialize the
supertokens-auth-react
SDK in your Vue app'smain.ts
file. This will provide session management across your entire application./main.tsimport { createApp } from "vue";
import * as SuperTokens from "supertokens-auth-react";
import * as EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
import App from "./App.vue";
import router from "./router";
SuperTokens.init({
appInfo: {
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
websiteDomain: "<YOUR_WEBSITE_DOMAIN>",
apiBasePath: "/auth",
websiteBasePath: "/auth"
},
recipeList: [
EmailPassword.init(),
Session.init(),
]
});
const app = createApp(App);
app.use(router);
app.mount("#app");
important
SuperTokens does not support pre-built UI for mobile frameworks. Please refer to the setup guide for custom UI to integrate SuperTokens in your app.
#
3) Setup Routing to show the login UI- ReactJS
- Angular
- Vue
- Mobile
Update your angular router so that all auth related requests load the auth
component
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
const routes: Routes = [
{
path: "auth",
loadChildren: () => import("./auth/auth.module").then((m) => m.AuthModule),
},
{ path: "**", loadChildren: () => import("./home/home.module").then((m) => m.HomeModule) },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
Update your Vue router so that all auth related requests load the AuthView
component
import { createRouter, createWebHistory } from "vue-router";
import HomeView from "../views/HomeView.vue";
const router = createRouter({
history: createWebHistory(import.meta.env.BASE_URL),
routes: [
{
path: "/",
name: "home",
component: HomeView,
},
{
path: "/auth/:pathMatch(.*)*",
name: "auth",
component: () => import("../views/AuthView.vue"),
},
],
});
export default router;
important
SuperTokens does not support pre-built UI for mobile frameworks. Please refer to the setup guide for custom UI to integrate SuperTokens in your app.
#
4) View the login UIYou can view the login UI by visiting /auth
. You can also see all designs of our pre built UI, for each page on this link.
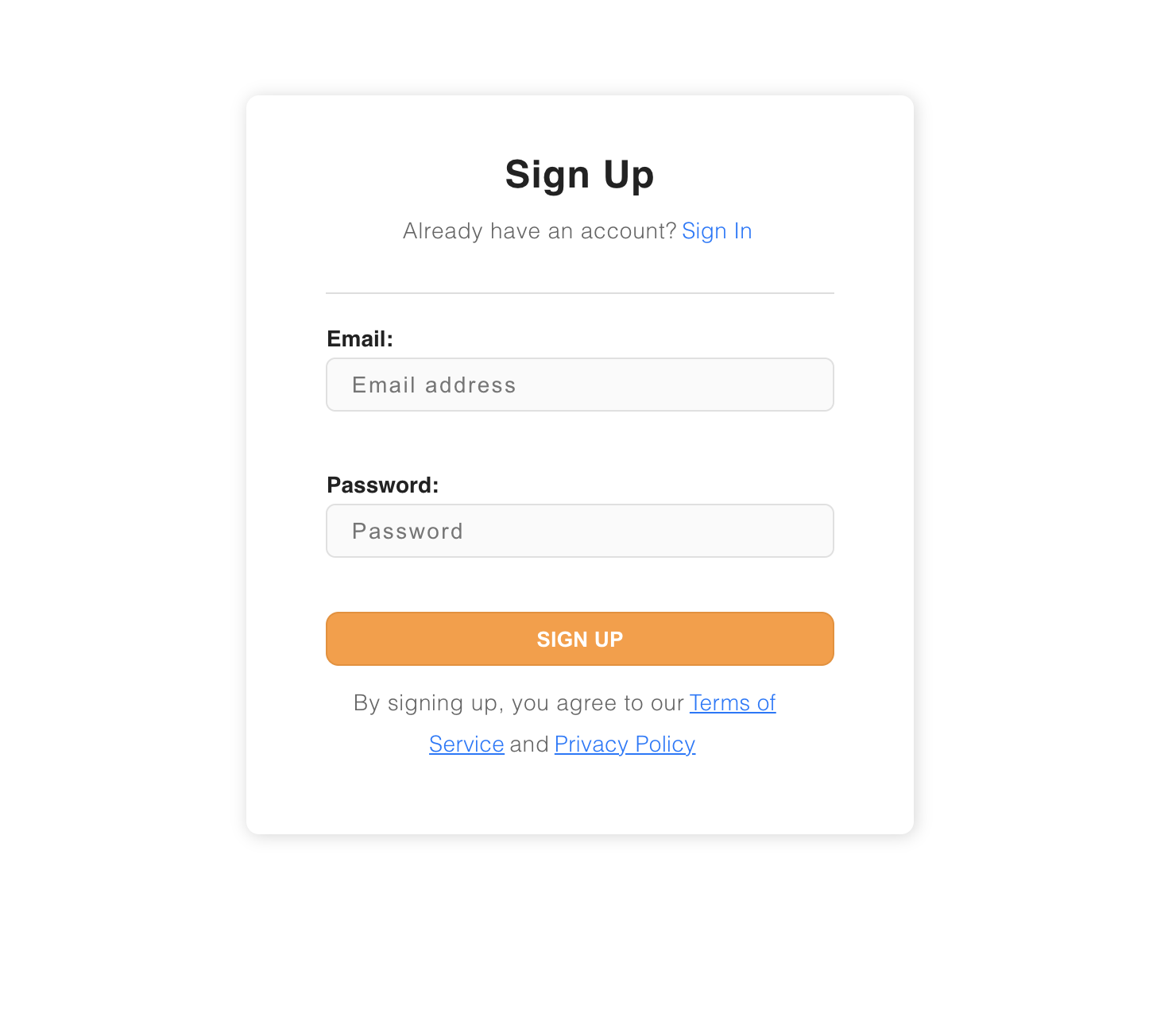
At this stage, you've successfully integrated your website with SuperTokens. The next section will guide you through setting up your backend.