Customising each form field
#
Modify Labels and Placeholder TextIf you would like to modify the fields in the login widget, by changing UI labels or placeholder text, you can do so by modifying the formFields
property when initializing SuperTokens on the frontend.
- ReactJS
- Angular
- Vue
Important
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "customFieldName",
placeholder: "Custom value"
}]
}
}
}),
Session.init()
]
});
Important
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "customFieldName",
placeholder: "Custom value"
}]
}
}
}),
Session.init()
]
});
import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "customFieldName",
placeholder: "Custom value"
}]
}
}
}),
Session.init()
]
});
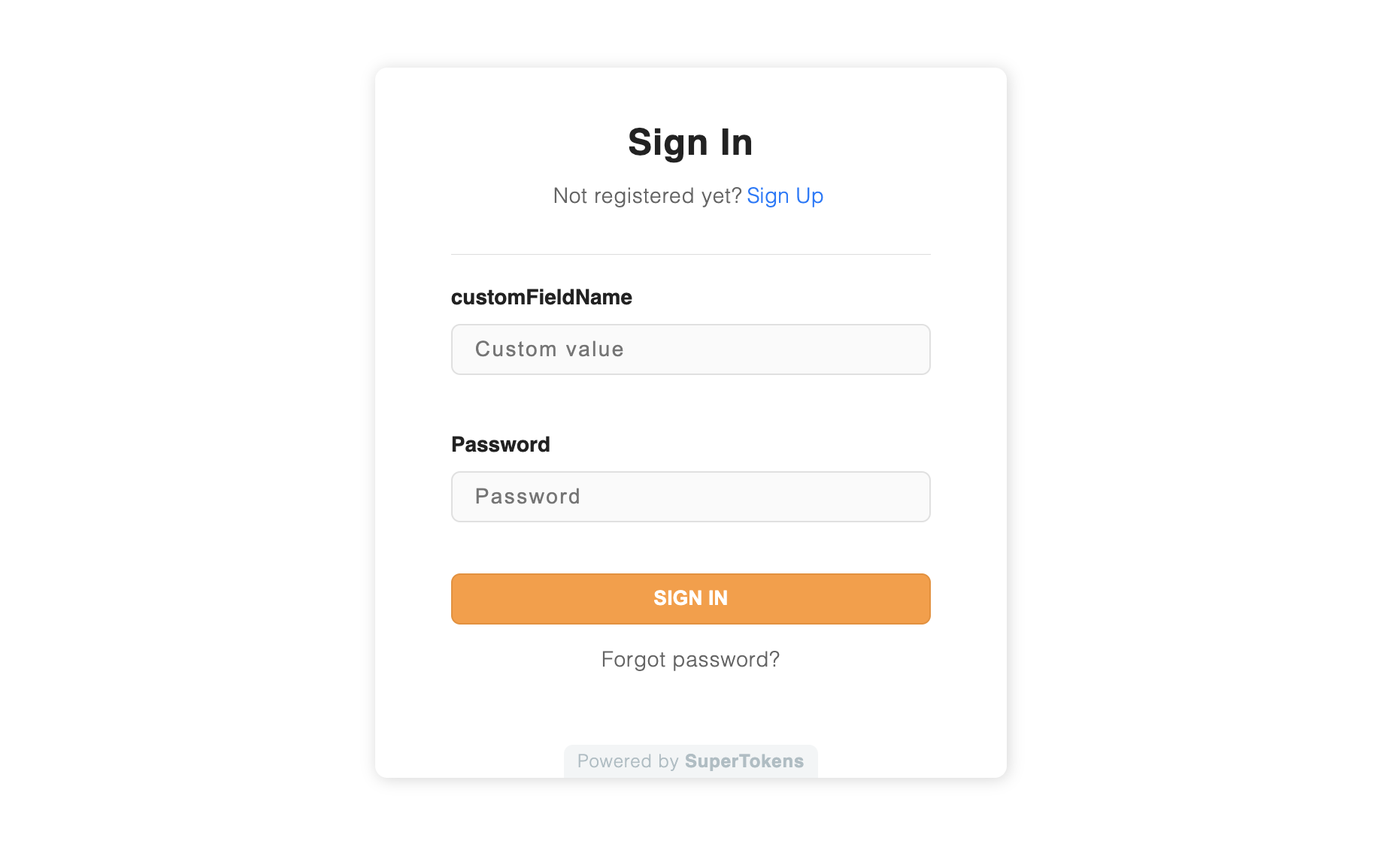
#
Setting Default ValuesTo fill in the form fields with preset values in the login widget, add a getDefaultValue
option to the formFields
config when initializing SuperTokens on the frontend.
- ReactJS
- Angular
- Vue
Important
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "Your Email",
getDefaultValue: () => "john.doe@gmail.com"
}]
}
}
}),
Session.init()
]
});
Important
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "Your Email",
getDefaultValue: () => "john.doe@gmail.com"
}]
}
}
}),
Session.init()
]
});
import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "Your Email",
getDefaultValue: () => "john.doe@gmail.com"
}]
}
}
}),
Session.init()
]
});
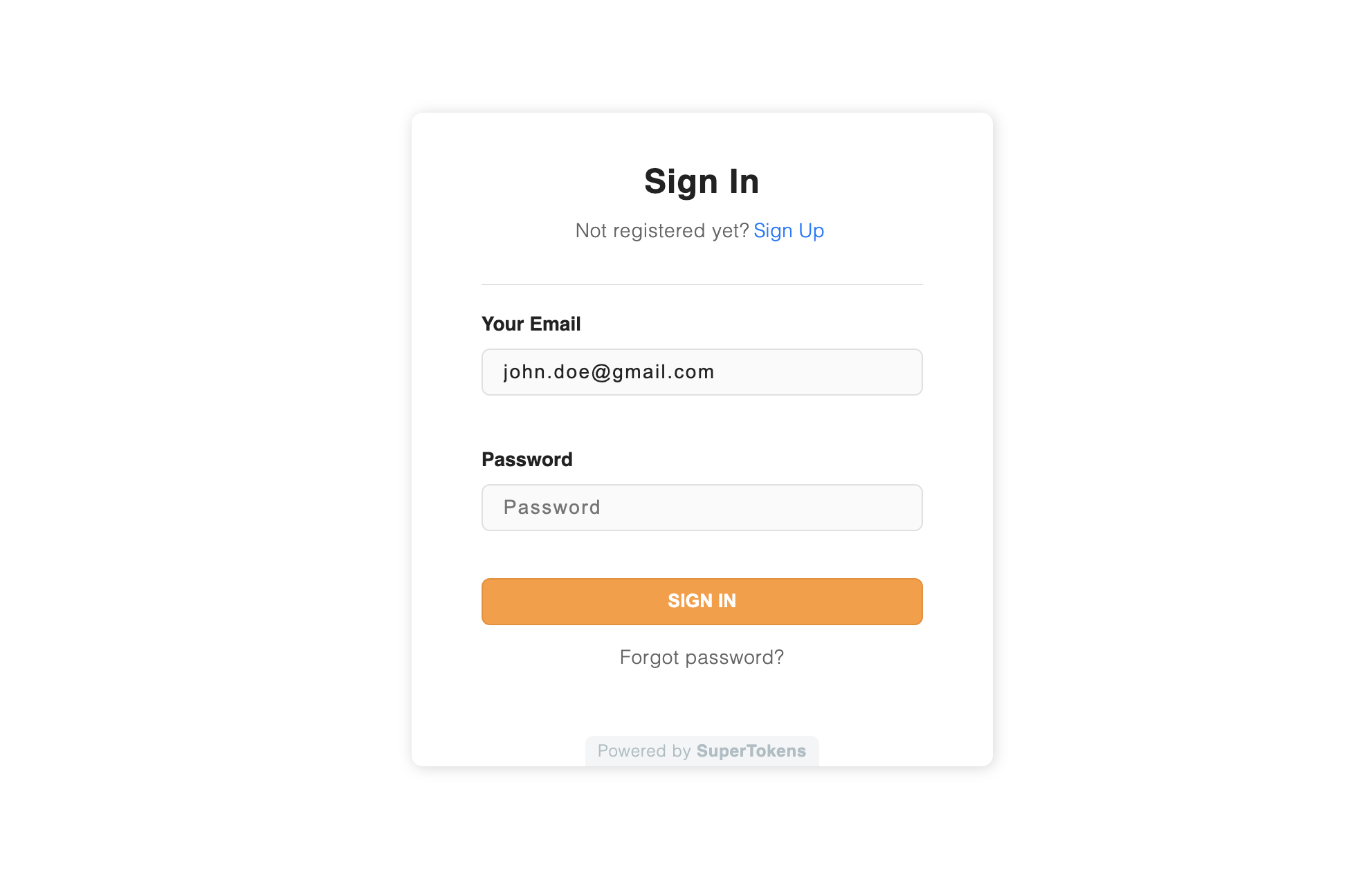
important
The return value of getDefaultValue
function must be a string
#
Changing Optional Error MessageWhen you try to submit login form without filling in required / non-optional fields, the SDK will, by default, show an error stating that the Field is not optional
. You can customize this error message with nonOptionalErrorMsg
property in the formField config.
Let's see how to achieve it.
- ReactJS
- Angular
- Vue
Important
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "Your Email",
placeholder: "Email",
nonOptionalErrorMsg: "Please add your email"
}]
}
}
}),
Session.init()
]
});
Important
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "Your Email",
placeholder: "Email",
nonOptionalErrorMsg: "Please add your email"
}]
}
}
}),
Session.init()
]
});
import SuperTokens from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
EmailPassword.init({
signInAndUpFeature: {
signInForm: {
formFields: [{
id: "email",
label: "Your Email",
placeholder: "Email",
nonOptionalErrorMsg: "Please add your email"
}]
}
}
}),
Session.init()
]
});
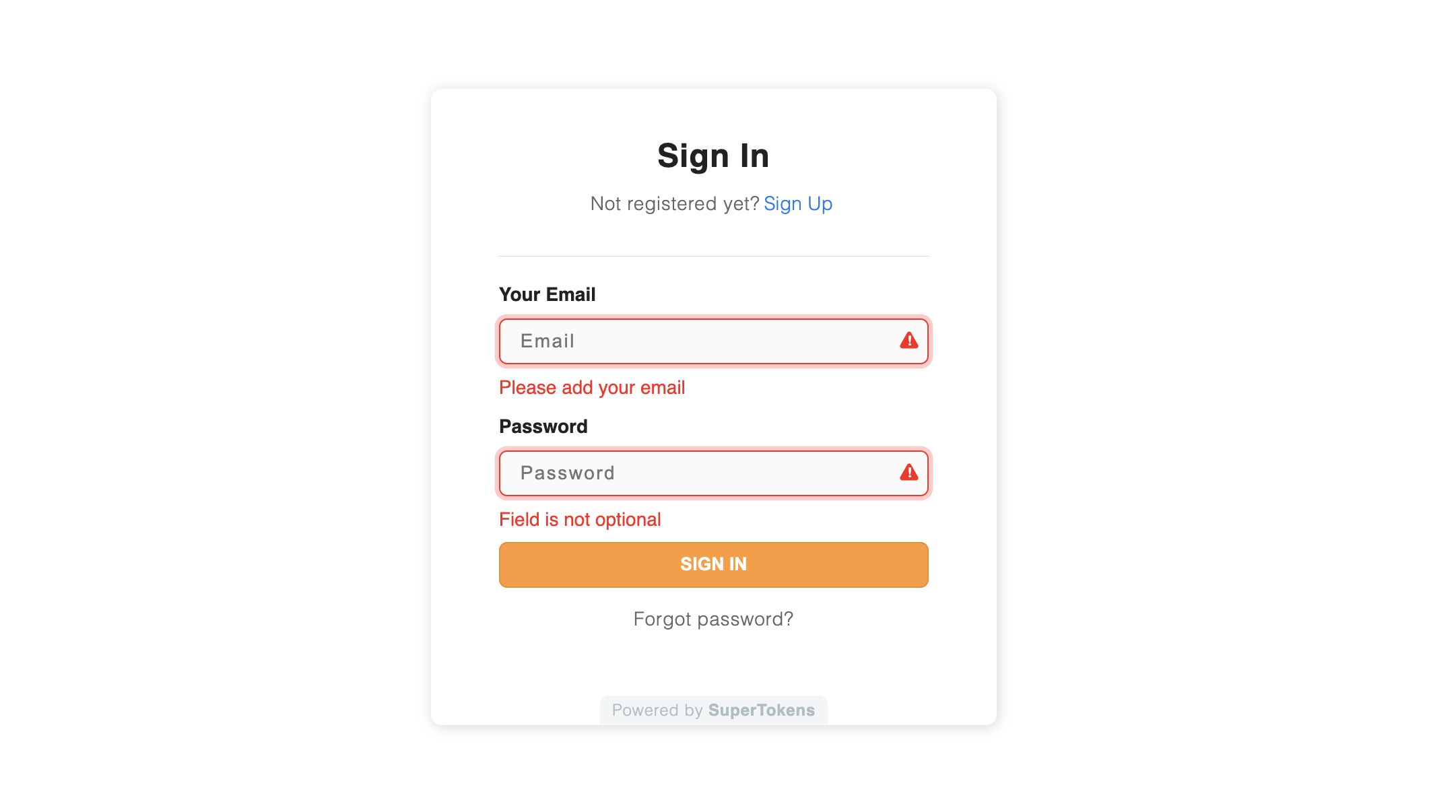
Observe how the password
field displays the standard error message because a custom message wasn't assigned using nonOptionalErrorMsg
for that field.
tip
To display an error message for required/non-optional fields, make use of the nonOptionalErrorMsg
property.
For complex validations of fields, make use of field validators.